Introduction
Have you ever been frustrated because your Python programs in VS Code always save in the root folder of your GitHub project, even if the program is in a subfolder? You’re not alone. I’ve encountered this problem too, and I couldn’t find a solution within VS Code’s settings.
Solution
The solution is within Python itself.
Python has a built-in variable called __file__
that refers to the path of the current Python file. By using this with os.path.join()
, you can ensure that your file will be saved in the same directory as your program, not in the root folder.
The os.path.join()
function in Python is a smart way to stick together pieces of a file path. It knows how to correctly use the right kind of slash (/
or \
) depending on your computer’s operating system. So, if you have a folder named “folder” and a file named “file.txt”, os.path.join("folder", "file.txt")
will give you the correct full path: “folder/file.txt”. It’s a handy tool for dealing with file paths in Python.
Here’s a simple demonstration of how to incorporate it. The code is available here for download.
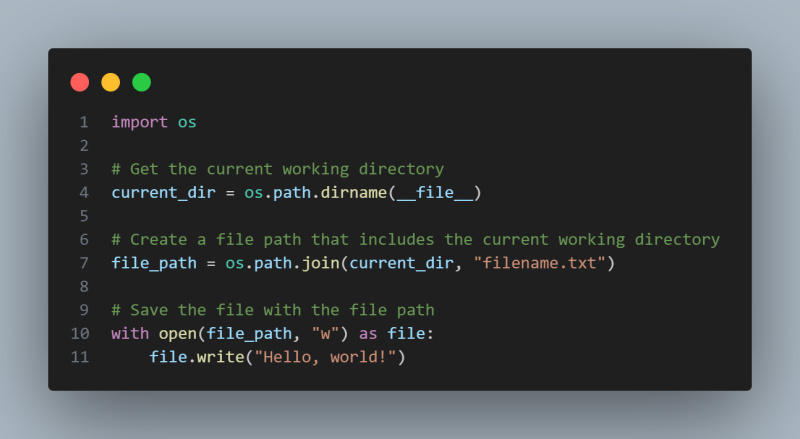
Software Overview
The program performs the following steps:
- Get the Current Working Directory: The program uses the
os.path.dirname(__file__)
command to get the directory of the current Python script. - Create a File Path: The program uses the
os.path.join(current_dir, "filename.txt")
command to create a file path that includes the current working directory and the filename “filename.txt”. - Save the File: The program opens the file with the created file path in write mode. If the file does not exist, it is created. If it does exist, it is overwritten. The program then writes the string “Hello, world!” to the file.
I hope this solution helps others who have encountered the same problem. If you find this useful, please let me know in the comments below!
One Reply to “How to Save Python Files in the Same Directory in VS Code”