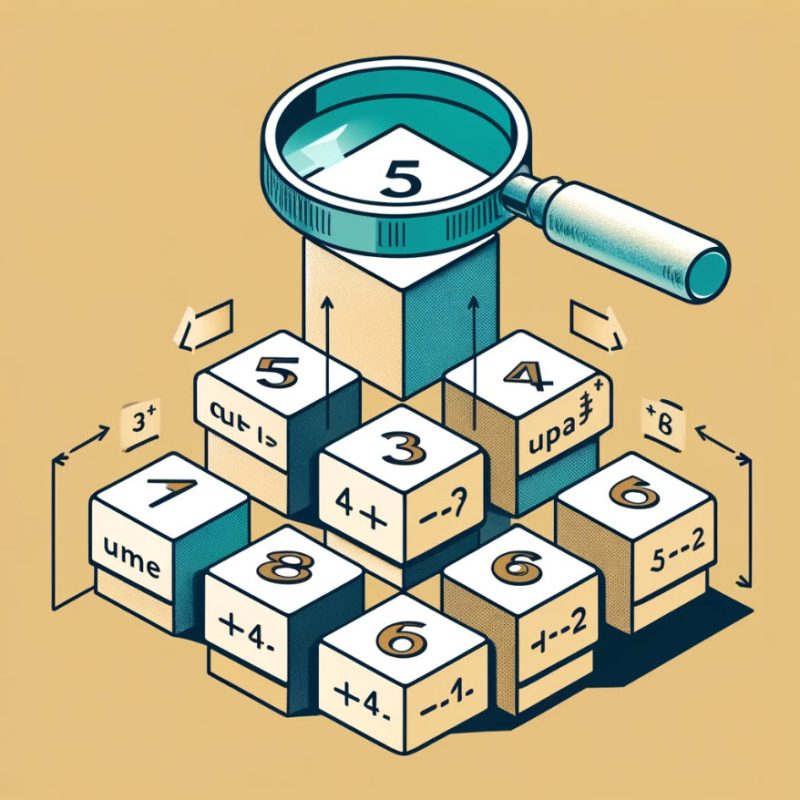
Table of Contents
Challenge Description:
You are provided with a list of n
non-negative integers. Your task is to write a program that performs the following operations:
- Query Mode:
- The program should first ask the user if they want to perform queries or updates:
Enter 'Q' for query and 'U' for update
. - If the user enters
Q
, prompt them to enter a range[l, r]
(1-based index) and then output the sum of elements from indexl
tor
inclusive.
- The program should first ask the user if they want to perform queries or updates:
- Update Mode:
- If the user enters
U
, prompt them to enter an indexi
(1-based index) and a valuev
. Update the element at indexi
by addingv
to it (this is an increment operation, not set). - After each update, print the new full array.
- If the user enters
The challenge is designed to teach basic array manipulations and looping constructs, with a focus on handling user inputs and performing range queries and updates efficiently.
Example
Let’s consider an array with initial values: [5, 3, 8, 6, 2]
Inputs and Outputs:
- User selects
Q
and enters1 3
: Output should be16
(sum of the first three elements:5+3+8
) - User selects
U
and inputs3 4
(meaning add4
to the third element): Array updates to[5, 3, 12, 6, 2]
and output the new array. - User selects
Q
and enters2 4
: Output should be21
(sum of the elements from index 2 to 4:3+12+6
)
Constraints:
- The array has at most
100
elements, and all operations should be efficiently handled within these bounds.
Test Input:
5
5 3 8 6 2
Q
1 3
U
3 4
Q
2 4
Test Output:
16
5 3 12 6 2
21
Programming Concepts Addressed
This programming challenge covers several fundamental and intermediate programming concepts that are crucial for developing proficiency in any programming language, especially Python. Here are the key concepts addressed:
- Arrays (Lists in Python):
- Manipulation and Access: Understanding how to access and modify elements in an array is fundamental. This involves working with indices to access values, which in this challenge are manipulated based on user input.
- Dynamically Modifying Array Contents: Learning how to update elements at specific indices and reflect these changes immediately in the program’s output.
- Control Structures:
- Conditional Statements (
if
,elif
,else
): Used to handle different commands (queries and updates) and validate input types. - Loops (
while
,for
): Essential for repeatedly executing operations, such as prompting the user until a termination condition ('E'
for exit) is met. Afor
loop could also be used if summing manually withoutsum()
function.
- Conditional Statements (
- Functions:
- Defining and Calling Functions: The entire functionality could be encapsulated into functions to manage code better, improve readability, and reuse code.
- Input and Output Operations:
- Reading User Input: Using
input()
to get user commands and parameters for queries and updates. - Printing Output: Displaying results back to the user, which helps in understanding how outputs are managed in response to dynamic inputs.
- Reading User Input: Using
- Exception Handling (Not explicitly covered but recommended for extension):
- Error Checking: Implementing try-except blocks to manage user input errors (like entering a non-integer where one is expected).
- Data Validation:
- Checking Input Validity: Ensuring that the user inputs for indices and update values are within the valid range of the array and are of correct types.
- Algorithmic Thinking:
- Efficiency Considerations: While the basic operations are simple, thinking about efficient ways to handle large inputs or numerous operations can lead into discussions on algorithmic complexity and possibly more advanced data structures (like segment trees for range queries).
- Modular Arithmetic:
- Index Conversion: Converting between 1-based user input indices and 0-based Python indices is a practical introduction to off-by-one errors and modular arithmetic considerations.
These concepts are not only foundational for programming in Python but also applicable across other programming languages. This challenge provides a hands-on way to practice these skills in an integrated manner, promoting a deeper understanding of how different aspects of programming interact in software development.
Summary
This challenge tests basic understanding of arrays, iterative processing, and conditional operations, making it suitable for beginner-level programmers. It also opens the door to more complex data structures like segment trees for range queries and updates if used as a teaching example.
This challenge was written with the help of ChatGPT. If you see any errors I would appreciate if you let me know via the comments below. Thanks!