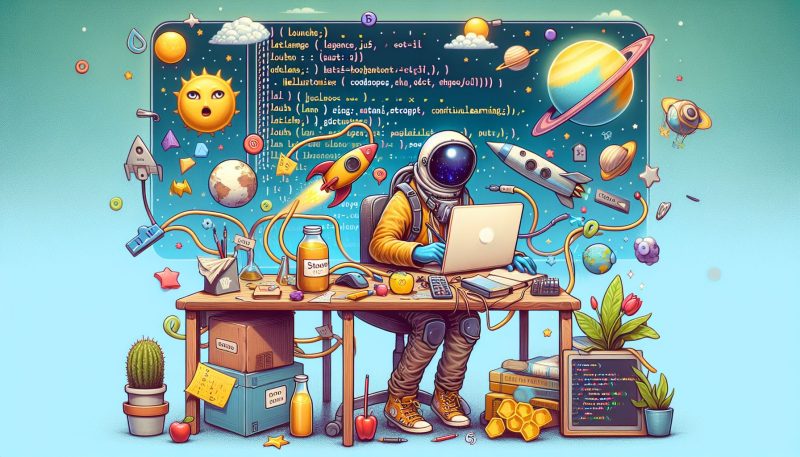
In a recent post I gave a programmatic solution to how to get Python to write files in the same folder as the Python file itself when working in VS Code. By default, Python programs in VS Code write files in the root folder of the project, not in the folder where the Python file is saved.
My solution involved the use of a built-in variable named “__file__
“, which together with some fancy path concatenation, achieved what I was hoping for.
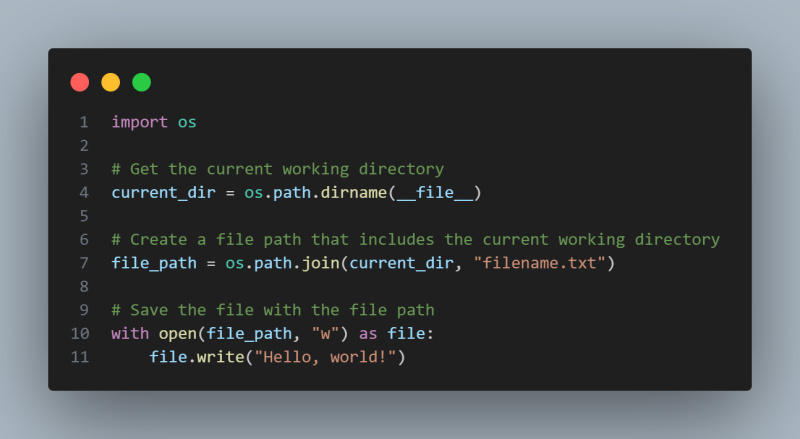
When I posted this on social media I got a response from @olkol@social.tchncs.de on Mastodon with an even better solution:
@pbeens
Adding
"cwd": "${fileDirname}",
to your vscode launch configuration should avoid this.
Perfect! Now I just needed to figure out how to do that!
Over to Microsoft’s Copilot with the question:
How do I add a line to my VS Code launch configuration? I want to add “cwd”:”${fileDirname}”,
To add a line to your VS Code launch configuration, you need to edit the launch.json
file under the .vscode
folder in your project. You can use one of the following techniques to open and modify the file:
- Use IntelliSense if your cursor is located inside the
configurations
array. Press theAdd Configuration
button to invoke snippet IntelliSense at the start of the array. - Choose
Add Configuration
option in theRun
menu. - Search for the
workbench.action.debug.selectandstart
command in the command palette and change the keybinding.
Once you have the launch.json
file open, you can add the line "cwd": "${fileDirname}"
to the configuration that you want to use. This will set the current working directory to the directory of the file being debugged.
That wasn’t going to work because I didn’t have a launch.json
file yet. Back to Copilot…
I don’t have a launch.json file.
No problem, you can create a launch.json file easily by following these steps:
- In VS Code, open the Run view by clicking the bug icon in the Activity Bar on the side of VS Code.
- Click the create a launch.json file link in the Run view.
- VS Code will try to automatically detect your debug environment, but if this fails, you will have to choose it manually from a list of available options.
- Once the launch.json file is created, you can add the line
"cwd": "${fileDirname}"
to the configuration that you want to use, as explained in my previous message.
I hope this helps you with your debugging. Happy coding! 😊
A few minutes to walk through that, then a quick test with my Python program…
# Create a new text file called hello.txt
with open("hello.txt", "w") as f:
# Write the string "Hello world" to the file
f.write("Hello world")
…in a subfolder got me the results I wanted—that is, the file hello.txt
in the same folder as my Python program!
Success! Social media for the win! (for once…)